Introduction to learning Docker
In the rapidly evolving tech landscape, Docker has become a transformative tool for building, deploying, and managing applications. For developers, system administrators, and DevOps professionals, mastering Docker is crucial for staying competitive in the tech industry. This complete guide on how to learn Docker will take you through essential Docker concepts and advanced practices. We’ll cover everything from Docker basics to practical examples, providing you with valuable resources and insights to enhance your Docker skills and streamline your application development process.
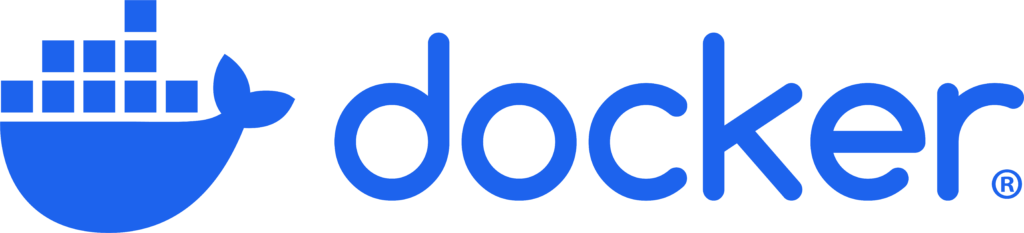
Table of Contents
RoadMap to learn Docker
Understanding the Basics of Docker
What is Docker?
- Build and Run Applications:
Docker is a powerful platform designed to simplify the way applications are developed, deployed, and executed. - Containers:
It uses small, lightweight containers to package your apps with everything they need. - Fast and Efficient:
Containers share the same operating system but run separately, making them faster and more resource-efficient than traditional virtual machines. - Easy to Manage:
Containers make it easier to manage and deploy your applications.
Key Docker Concepts
- Containers: The encapsulated environments where your applications run.
- Images: The blueprint for containers, containing everything needed to run an application, including the code, runtime, libraries, and dependencies.
- Docker Hub: A cloud-based registry where you can find and share Docker images.
- Dockerfile: A text file that contains instructions to build a Docker image.
- Docker Compose: A tool for managing applications with multiple Docker containers. It uses a docker-compose.yml file to define and configure various services, networks, and storage volumes, enabling you to set up and control complex applications with a single command
Docker Architecture
Docker operates on a client-server architecture, where the Docker client communicates with the Docker daemon. The Docker daemon is responsible for creating, managing, and distributing containers. The Docker client and daemon can run on the same system, or the client can connect to the daemon over a REST API using UNIX sockets or a network connection.
Docker’s architecture is built around the concept of containers, which package an application and its dependencies into a single, lightweight unit that can run consistently across different environments. Here’s an overview of Docker’s architecture:
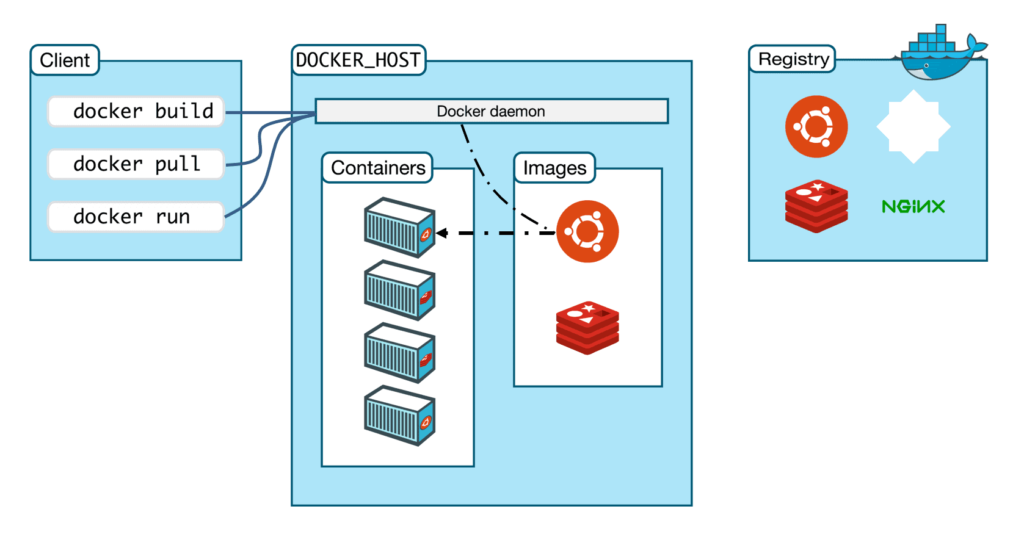
Docker Client:
- The Docker client is the primary way users interact with Docker. It sends commands to the Docker daemon using the Docker CLI (Command Line Interface).
- Common commands include docker build, docker pull, docker run, etc.
- The client communicates with the Docker daemon either on the same machine or remotely via REST API.
Docker Daemon (dockerd)
- The Docker daemon is the core service that manages Docker containers, images, networks, and storage volumes.
- It listens for API requests and manages Docker objects such as images, containers, networks, and volumes.
- The daemon is responsible for building, running, and distributing Docker containers.
Docker Images
- Docker images are read-only templates that contain the instructions for creating a container.
- They are built using a Dockerfile, which is a script containing a series of commands to assemble the image.
- Images can be stored in a Docker registry, such as Docker Hub, and are pulled by the Docker daemon when needed.
- Dockerhub https://hub.docker.com/
Docker Containers
- Containers are the runnable instances of Docker images. They are lightweight and contain everything needed to run an application.
- Containers are isolated from each other and the host system, but they can share resources like CPU, memory, and storage.
- You can start, stop, move, or delete containers using Docker commands.
Docker Registry
- A Docker registry is a repository for Docker images. Docker Hub is the most popular public registry, but private registries can also be set up.
- Registries allow users to share and distribute images. Docker pull/push commands are used to interact with the registry.
- Images are versioned and can be tagged to identify different stages or releases of an application.
Docker Networking
Docker provides various networking options to connect containers to each other and to external networks,including
- Bridge Networks: Default isolated networks for communication between containers on the same host.
- Host Networks: Containers share the host’s network stack for direct external access.
- None: Disables networking for the container, isolating it completely.
Docker Host
- The Docker Host is the physical or virtual machine where the Docker daemon runs. It provides the resources (CPU, memory, storage) needed to build, run, and manage containers.
- It can be a local machine, a cloud-based instance, or any system where Docker is installed and running.
Docker Commands
docker run
: Creates and starts a new container from an image.
Example: docker run image_name
docker pull
: Fetches a Docker image from a registry.
Example:docker pull image_name
docker ps
: Lists all running containers.
Example:docker ps
docker images
: Lists all available Docker images on your system.
Example:docker images
docker stop
: Stops a running container.
Example:docker stop container_id
docker start
: Starts a stopped container.
Example:docker start container_id
docker restart
: Stops and then starts a container.
Example:docker restart container_id
docker rm
: Removes one or more containers.
Example:docker rm container_id
docker rmi
: Removes one or more Docker images.
Example:docker rmi image_name
docker logs
: Displays the logs of a container.
Example:docker logs container_id
docker exec
: Runs a command inside a running container.
Example:docker exec container_id command
docker build
: Builds a Docker image from a Dockerfile.
Example:docker build -t image_name
Dockerfile
A Dockerfile is a text file that contains a series of instructions to build a Docker image.
It automates the process of creating a Docker image with your desired configuration, software, and dependencies, ensuring consistency across different environments.
Creating a Simple Dockerfile for a Basic Python Application
In this example, we’ll create a Dockerfile to run a basic Python application that prints “Hello, Docker!” to the console. This example will help you understand the essentials of Dockerfiles without needing additional configurations or files.
Create app.py
# app.py
print("Hello, Docker!")
Create the Dockerfile
# Use the official Python image from Docker Hub
FROM python:3.9-slim
# Set the working directory inside the container
WORKDIR /app
# Copy the Python script into the container
COPY app.py /app/
# Run the Python script
CMD ["python", "app.py"]
Explanation of the Dockerfile
- FROM python:3.9-slim: Specifies the base image, which is a lightweight Python 3.9 image.
- WORKDIR /app: Sets the working directory inside the container to /app.
- COPY app.py /app/: Copies the app.py file from your local directory to the /app directory in the container.
- CMD [“python”, “app.py”]: Defines the default command to run the Python script when the container starts.
Building and Running the Docker Image
In the directory containing the Dockerfile and app.py, run:
docker build -t hello-world-python .
This command builds an image named hello-world-python.
Run the Docker Container:
docker run hello-world-python
This command runs the container, which executes the Python script and prints “Hello, Docker!” to the console.
Docker Compose
Docker Compose simplifies managing multi-container applications by defining services, networks, and volumes in a single docker-compose.yml file. This makes deploying and managing complex setups easier and more consistent.
Key Concepts
Services: Containers performing specific functions. Defined in docker-compose.yml with configuration details like image, environment variables, and ports.
Networks: Facilitate communication between containers. Docker Compose creates a default network or you can define custom ones.
Volumes: Persist and share data between containers. Ensure data durability and manage application state.
Example docker-compose.yml
version: '3'
services:
web:
image: nginx:latest
ports:
- "8080:80"
networks:
- my-network
db:
image: postgres:latest
environment:
POSTGRES_DB: mydatabase
POSTGRES_USER: user
POSTGRES_PASSWORD: password
volumes:
- db-data:/var/lib/postgresql/data
networks:
- my-network
networks:
my-network:
driver: bridge
volumes:
db-data:
Common Commands
docker-compose up: Start and run your containers.
docker-compose down: Stop and remove containers, networks, and volumes.